Java Operator Precedence Table
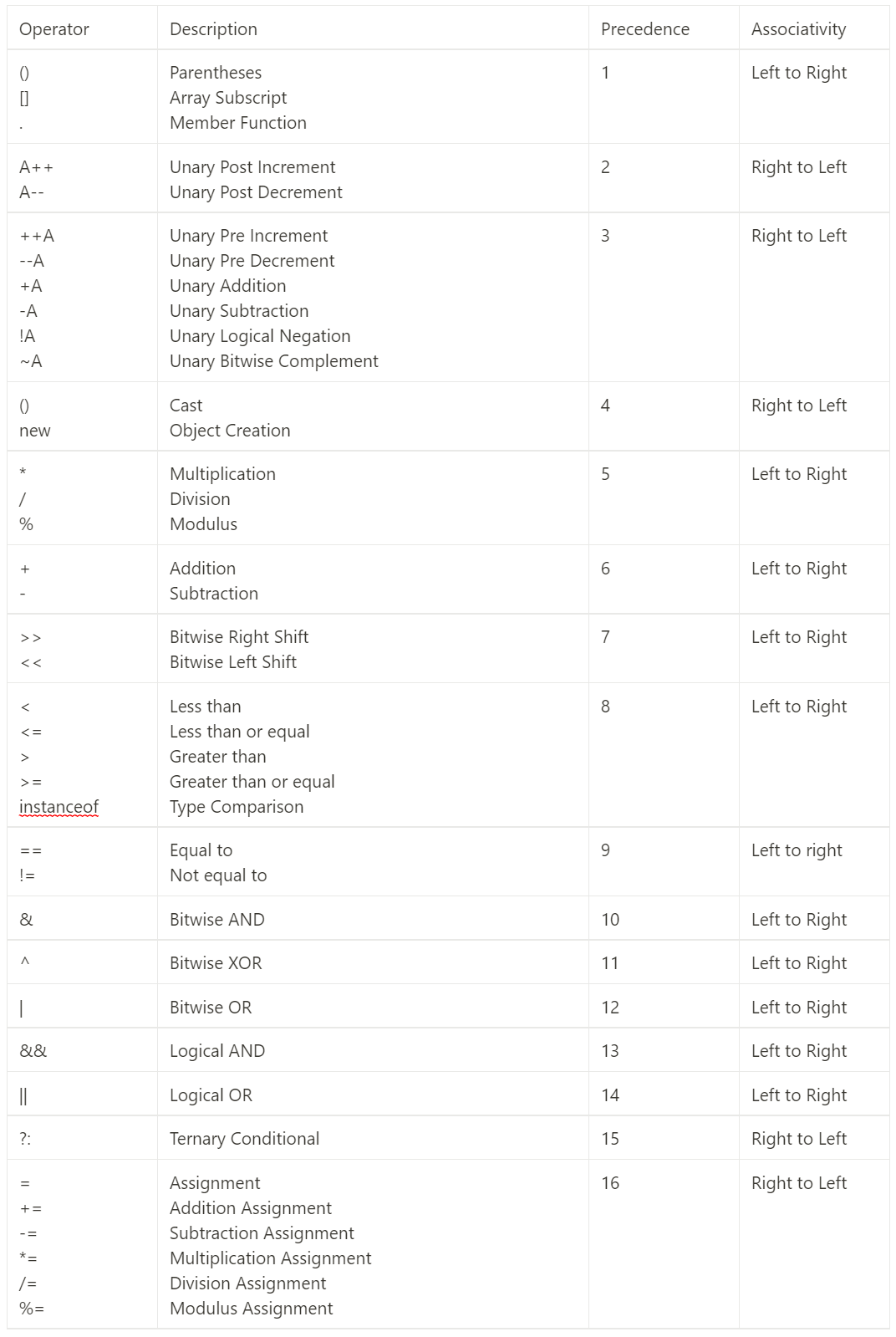
Definition of Operator Precedence
Operator precedence refers to the grouping of operators in a complex expression based on their priority. This does not mean that the operators are evaluated in the order of precedence.
Example
Consider the following expression, in which it is not clear whether the computer should evaluate a > 0
or 0 && b
first:
int a = 1, b = 1;
a > 0 && b - ++a == 1
Based on the Java Operator Precedence Table, the expression is first grouped as follows:
a > 0 && b - ++a == 1
a > 0 && b - (++a) == 1
a > 0 && (b - (++a)) == 1
a > 0 && (b - (++a)) == 1
(a > 0) && (b - (++a)) == 1
(a > 0) && ((b - (++a)) == 1)
The expression is now evaluated from left to right based on the Logical AND operator:
(1 > 0) && ((b - (++a)) == 1)
true && ((b - (++a)) == 1)
true && ((b - (++1)) == 1)
The pre-increment operator is now applied, which increments the value of the operand by 1 before any other operation is performed:
true && ((b - 2) == 1)
true && ((1 - 2) == 1)
true && (-1 == 1)
true && false
false
Increment and Decrement Operators
Operator | Description |
---|---|
++A | Pre-increment: increases the value of operand A by 1, and then returns the new value |
A++ | Post-increment: returns the value of operand A, and then increases its value by 1 |
--A | Pre-decrement: decreases the value of operand A by 1, and then returns the new value |
A-- | Post-decrement: returns the value of operand A, and then decreases its value by 1 |
Logical OR Operator
The logical OR operator (||
) evaluates the operands from left to right. If the left-hand operand is true
, the right-hand operand is not evaluated, and the result of the operation is true
. If the left-hand operand is false
, the right-hand operand is evaluated, and the result of the operation is the value of the right-hand operand.
For example:
(1==1)||(1==2)
boolean a = true;
boolean b = false;
boolean c = a || b; // c is true, b is not evaluated
Conclusion
Operator precedence is a way to group operators in an expression based on their precedence level. This helps to determine the order of evaluation when there are multiple operators in an expression. However, it does not dictate the actual order of operations. The order of operations is determined by the individual operator's characteristics and the operands involved.
댓글 없음:
댓글 쓰기